Recently I worked on a simple kiosk for a new exhibit in the library, Yasak/Banned: Political Cartoons from Late Ottoman and Republican Turkey. The interface presents users with three videos featuring the curators of the exhibit that explain the historical context and significance of the items in the exhibit space. We also included a looping background video that highlights many of the illustrations from the exhibit and plays examples of Turkish music to help envelop visitors into the overall experience.
With some of the things I’ve built in the past, I would setup different section of an interface to view videos, but in this case I wanted to keep things as simple as possible. My plan was to open each video in an overlay using fancybox. However, I didn’t want the looping background audio to interfere with the curator videos. We also needed to include a ‘play/pause’ button to turn off the background music in case there was something going on in the exhibit space. And I wanted all these transitions to be as smooth as possible so that the experience wouldn’t be jarring. What seemed reasonably simple on the surface proved a little more difficult than I thought.
After trying a few different approaches with limited success, as usual stackoverflow revealed a promising direction to go in — the key turned out to be the .animate jQuery method.
The first step was to write functions to ‘play/pause’ – even though in this case we’d let the background video continue to play and only lower the volume to zero. The playVid function sets the volume to 0, then animates it back up to 100% over three seconds. pauseVid does the inverse, but more quickly.
function playVid() {
vid.volume = 0;
$('#myVideo').animate({
volume: 1
}, 3000); // 3 seconds
playBtn.style.display = 'none';
pauseBtn.style.display = 'block';
}
function pauseVid() {
// really just fading out music
vid.volume = 1;
$('#myVideo').animate({
volume: 0
}, 750); // .75 seconds
playBtn.style.display = 'block';
pauseBtn.style.display = 'none';
}
The play and pause buttons, which are positioned on top of each other using CSS, are set to display or hide in the functions above. The are also set to call the appropriate function using an onclick event. Their markup looks like this:
<button onclick="playVid()" type="button" id="play-btn">Play</button>
<button onclick="pauseVid()" type="button" id="pause-btn">Pause</button>
Next I added an onclick event calling our pause function to the link that opens our fancybox window with the video in it, like so:
<a data-fancybox-type="iframe" href="https://my-video-url" onclick="pauseVid();"><img src="the-video-thumbnail" /></a>
And finally I used the fancybox callback afterClose to ramp up the background video volume over three seconds:
afterClose: function () {
vid.volume = 0;
$('#myVideo').animate({
volume: 1
}, 3000);
playBtn.style.display = 'none';
pauseBtn.style.display = 'block';
},
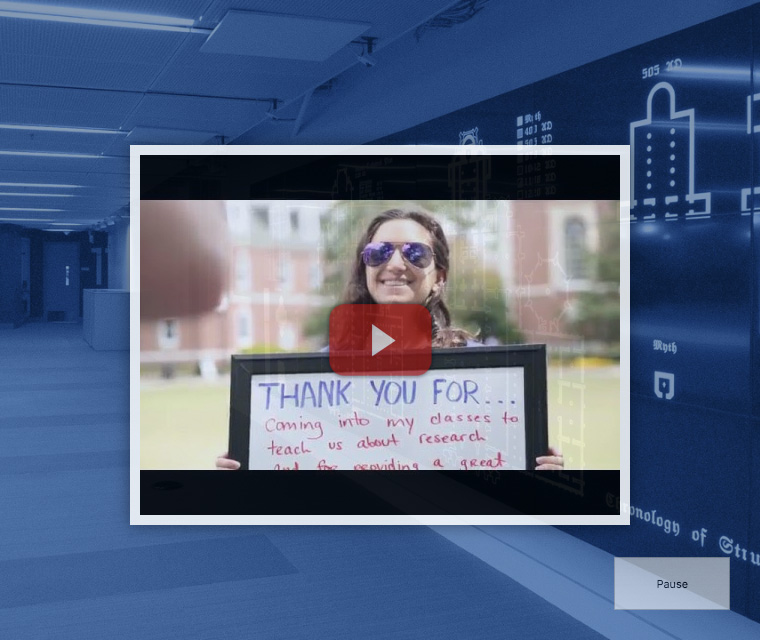
You can view a demo version and download a zip of the assets in case it’s helpful. I think the final product works really well and I’ll likely use a similar implementation in future projects.